In this guide, we will closely look at Kubernetes (K8s) Jobs - what they are, their types, how to use them, handling errors related to K8s Jobs, and much more.
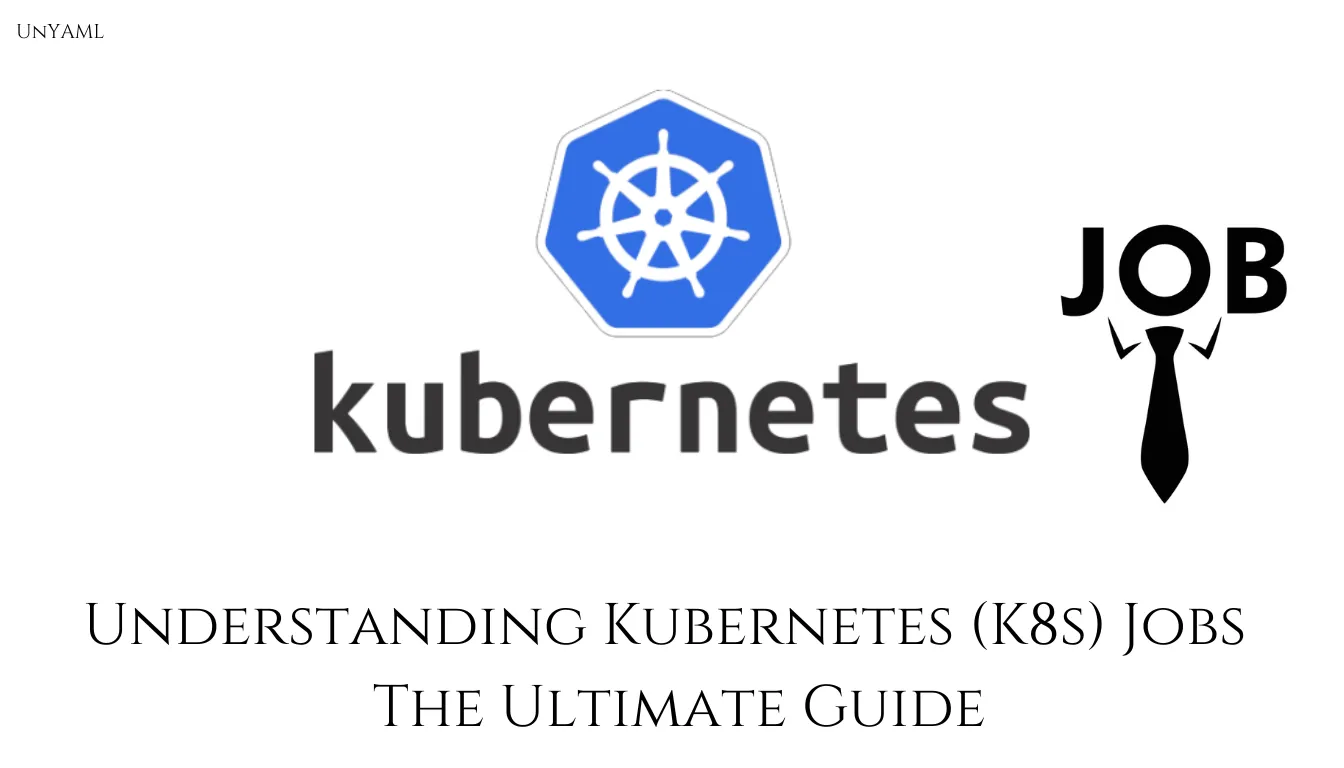
If you're curious about Kubernetes and wondering what those "Jobs" are all about, you've come to the right place!
In this Kubernetes guide, we'll break down the concept of Jobs in Kubernetes in simple terms, so you can understand how they fit into the larger picture of container orchestration.
Whether you're a beginner or just looking to expand your Kubernetes knowledge, let's dive in and demystify the role of Jobs in this powerful technology.
What are Kubernetes Jobs?
In Kubernetes, a "Job" is a resource used to manage short-lived, non-replicated tasks or batch jobs. It's designed for situations where you need to run a task once or a limited number of times, ensuring that it is completed successfully.
Think of it as a way to perform specific workloads or computations reliably within a Kubernetes cluster without the need for continuous monitoring.
Jobs are especially useful for scenarios like data processing, cron-like tasks, and one-time jobs where you want Kubernetes to handle the execution, scaling, and cleanup automatically.
When you create a Job, Kubernetes ensures that the task is executed to completion, and if it fails, it can be retried a specified number of times.
Also Read: Working with Kubernetes Cluster using Kubeadm
Types Of Kubernetes Jobs
Let's look at some different types of Kubernetes Jobs.
1. Single Job
This is the simplest type of Job, where you want to run a task once and ensure it is completed successfully.
Here's an example.
Let's say you are trying to run a one-time database migration when deploying a new version of your application.
This is how you can create a job for accomplishing the above requirement:
apiVersion: batch/v1
kind: Job
metadata:
name: database-migration
spec:
template:
spec:
containers:
- name: migration-container
image: your-migration-image
backoffLimit: 1 # Number of retries
Also Read: Everything to Know About Port Forwarding in Kubernetes
2. Scheduled Job (CronJob)
CronJobs are used when you want to run a Job on a schedule, similar to a cron job in a traditional Unix environment.
Here's a scenario where CronJon in Kubernetes can come in handy - running a daily backup of your application's data.
apiVersion: batch/v1beta1
kind: CronJob
metadata:
name: daily-backup
spec:
schedule: "0 0 * * *" # Schedule in cron format (midnight every day)
jobTemplate:
spec:
template:
spec:
containers:
- name: backup-container
image: your-backup-image
restartPolicy: OnFailure
3. Parallel Job (Parallelism)
Parallel Jobs allows you to run multiple pods (tasks) in parallel to complete a job quickly.
You can use Parallel Jobs in Kubernetes for processing a large number of images concurrently.
apiVersion: batch/v1
kind: Job
metadata:
name: image-processing
spec:
parallelism: 5 # Number of pods running in parallel
completions: 10 # Number of pods to complete before considering the Job as done
template:
spec:
containers:
- name: processing-container
image: your-processing-image
backoffLimit: 2 # Number of retries
These examples illustrate different types of Kubernetes Jobs to handle tasks that range from one-time jobs to scheduled and parallelized tasks.
Creating and configuring a Job in Kubernetes involves specifying the Job's characteristics such as labels, the pod template, and the pod selector. Also Read: Kubernetes Nodes vs. Pods vs Cluster
How to Configure a Job in Kubernetes?
In this section, we will closely look at how to create/configure a Kubernetes Job.
1. Define a YAML Configuration File
Create a YAML configuration file (my-job.yaml
for instance) to define the Job. In this file, you'll specify the Job's metadata, pod template, and other details.
2. Metadata for the Kubernetes Job
apiVersion: batch/v1
kind: Job
metadata:
name: my-job
labels:
app: my-app
apiVersion and kind define the Kubernetes resource type as a Job.
metadata contains information about the Job, including its name and labels. labels can help you identify and organize Jobs within your cluster.
Also Read: Understanding Kubernetes Taints & Tolerations
3. Pod Template for the K8s Job
The pod template defines the specifications for the pods that the Job will create. It includes details like the container image to use, a command to run, and environment variables.
spec:
template:
metadata:
labels:
app: my-app
spec:
containers:
- name: my-container
image: my-image:1.0
command: ["my-command"]
args: ["arg1", "arg2"]
restartPolicy: OnFailure
- spec.template.metadata.labels sets labels for the pods created by the Job. These labels can be used for pod selection and identification.
- spec.template.spec.containers specifies the container to run in the pod, along with the image, command, and arguments.
- restartPolicy determines what happens when a pod in the Job completes. Use "OnFailure" to ensure that only failing pods are restarted.
Also Read: Understanding RelicaSets in Kubernetes
4. Pod Selector
A Job uses a pod selector to identify which pods it should manage. The selector should match the labels you defined earlier.
spec:
selector:
matchLabels:
app: my-app
spec.selector.matchLabels specifies the labels that the Job uses to select pods. In this example, it selects pods with the label "app: my-app."
5. Backoff Limit
You can set the backoff limit to control how many times Kubernetes retries the Job if it fails. By default, it's set to 6.
spec:
backoffLimit: 3
Also Read: How to Fix CrashLoopBackoff Error in Kubernetes?
6. Apply the Configuration
Apply the configuration to your Kubernetes cluster using the kubectl apply command:
kubectl apply -f my-job.yaml
7. Check Job Status
Monitor the Job's status using kubectl get jobs and kubectl describe job my-job commands. You can also check the status of individual pods using kubectl get pods.
That's it!
You've now created and configured a Kubernetes Job. It will create pods based on the specified pod template and manage them according to your settings.
This approach (creating Kubernetes Jobs) allows you to automate tasks, ensure reliable execution, and maintain the desired state of your applications within the cluster.
After creating a Kubernetes Job and understanding its fundamentals, let's look at how you can update your job by making changes in the file and some other operations related to Kubernetes jobs.
Managing Kubernetes Jobs: Configuration Updates, Post-Deployment Execution, and Custom Pod Selection
Changing the configuration file of a Kubernetes Job involves making updates to the existing YAML file or creating a new one with the desired changes.
Below, the instructions are provided on how to change a Job's configuration file, run a Job after deployment, and specify your own pod selector:
Changing the Configuration File of a Kubernetes Job
-
Open the existing Job's YAML configuration file (my-job.yaml,for instance) in a text editor.
-
Modify the sections you want to change. This can include the Job's metadata, pod template, labels, or any other relevant fields.
-
Save the changes to the configuration file.
-
Apply the updated configuration to the Kubernetes cluster using the kubectl apply command:
kubectl apply -f my-job.yaml
This will update the Job with the new configuration.
Also Read: How to Use Terraform Apply Command?
Running a Kubernetes Job After Deployment
If you want to run a Job after deploying it to the cluster, you can create and apply the Job configuration separately.
Let's say you have a Deployment and you want to run a Job after deploying it:
-
Create a Job YAML configuration file (e.g., run-job-after-deployment.yaml) with your desired Job configuration.
-
Apply the Job configuration using kubectl apply
This way, the Job will be created and run after the Deployment is already in place.
Also Read: How to Keep Docker Running Indefinitely?
Specifying Your Own Pod Selector with Kubernetes Jobs
By default, a Kubernetes Job uses its own auto-generated pod selector to manage pods.
However, if you want to specify your own pod selector labels, you can do so in the Job's configuration.
Here's an example:
apiVersion: batch/v1
kind: Job
metadata:
name: my-job
spec:
selector:
matchLabels:
custom-selector: my-selector
template:
metadata:
labels:
custom-selector: my-selector
spec:
containers:
- name: my-container
image: my-image:1.0
In this example, you can specify your own pod selector using the selector.matchLabels field.
Both the Job and the pod template have the label custom-selector: my-selector.
This ensures that the Job selects and manages pods with this specific label, providing more control over pod selection.
Well, it's time to know how you can delete these jobs and perform a cleanup.
Also Read: How to Cleanup Docker Resources?
How to Delete a Kubernetes Job/ Perform a Cleanup?
Deleting or cleaning up Kubernetes Jobs is an important task to manage cluster resources efficiently.
Here's how to delete a Kubernetes Job manually and configure automatic cleanup for finished Jobs.
Manual Deletion of a Kubernetes Job
To manually delete a Kubernetes Job, you can use the kubectl delete command.
Here's how:
kubectl delete job <job-name>
Replace <job-name>
with the actual name of the Job you want to delete. This command will initiate the deletion process for the specified Job.
If the Job has associated pods, those pods will also be deleted.
Also Read: When and How to Use Kubectl Delete Deployment?
Automatic Cleanup for Finished Kubernetes Jobs
You can configure Kubernetes to automatically clean up finished Jobs using the ttlSecondsAfterFinished field. This field sets a time-to-live (TTL) duration for completed Jobs.
After this duration elapses, the Job and its pods will be automatically cleaned up.
Here's how to configure automatic cleanup for finished Jobs:
apiVersion: batch/v1
kind: Job
metadata:
name: my-job
spec:
template:
spec:
containers:
- name: my-container
image: my-image:1.0
restartPolicy: Never
ttlSecondsAfterFinished: 86400 # Specify the TTL duration in seconds (e.g., 24 hours)
In this example, ttlSecondsAfterFinished is set to 86400 seconds, which equals 24 hours. This means that after the Job is completed, it and its pods will be automatically deleted 24 hours later.
You can adjust the ttlSecondsAfterFinished value to match your specific cleanup requirements.
By implementing automatic cleanup, you ensure that completed Jobs and their associated pods do not clutter your cluster's resources and are automatically removed after the specified TTL duration, helping to keep your cluster clean and efficient.
But you know, that errors and failures are a part of learning Kubernetes, so let's see some of the ways of handling those.
Handling Job Failures & Concurrency
Handling Job failures and managing concurrency are crucial aspects when working with Kubernetes Jobs to ensure the reliability and efficiency of your workloads.
Here's how to handle Job failures and concurrency effectively.
1. Retry Policies
Kubernetes allows you to define how many times a Job should be retried upon failure. You can set the backoffLimit field in the Job's specification.
For example, backoffLimit: 3 will retry the Job up to three times.
Ensure that your Job's container and application inside it handle failures gracefully. Implement proper error handling, logging, and exit codes to indicate success or failure.
Also Read: How to Fix OOMKilled Error in Kubernetes?
2. Notifications
Set up monitoring and alerting for your Jobs. Tools like Prometheus and Grafana can help you monitor Job statuses and send alerts when failures occur.
3. Logs and Debugging
Utilize Kubernetes logs to troubleshoot Job failures. You can access pod logs using kubectl logs <pod-name>
.
Implement debug information within your containers to assist in diagnosing issues.
Also Read: Understanding Kubernetes Events
Managing Concurrency
1. Parallelism
Kubernetes Jobs allow you to specify the number of pods (tasks) running concurrently using the parallelism field. Adjust this value based on your cluster's capacity and resource requirements.
spec:
parallelism: 5 # Example: Run 5 pods in parallel
2. Resource Requests and Limits
Define resource requests and limits for your Job containers to control resource usage.
This helps prevent resource contention and ensures that Jobs don't consume more than their fair share.
spec:
template:
spec:
containers:
- name: my-container
resources:
requests:
cpu: "0.5"
memory: "512Mi"
limits:
cpu: "1"
memory: "1Gi"
Also Read: Horizontal vs Vertical Scaling
3. Scaling
If your Jobs have variable workloads, consider using Horizontal Pod Autoscaling (HPA) to dynamically adjust the number of pods based on demand.
By addressing these aspects, you can effectively handle Job failures and manage concurrency in your Kubernetes cluster, ensuring that Jobs are executed reliably and efficiently while maintaining cluster stability.
With this, you have reached the end of the blog. Let's summarize it with a small conclusion.
Final Words: Kubernetes Jobs
In this guide, we have explored various job types, including Single Jobs, Scheduled Jobs (CronJobs), and Parallel Jobs, each suited to different needs.
Job patterns and templates make it easier to create and reuse configurations, streamlining your workload management.
We walked through creating and configuring Jobs, diving deep into elements like Job labels, pod templates, and custom pod selectors.
Additionally, you learned how to change Job configurations, run them post-deployment, and manage Job cleanup automatically.
Job completion and handling failures are critical aspects, ensuring tasks are completed reliably.
By managing concurrency and resource usage, Kubernetes Jobs becomes even more effective in your cluster. These insights will empower you to master Kubernetes Jobs for streamlined task execution in your containerized environment.